Spiral Matrix
Problem
Given an m x n
matrix
, return all elements of the matrix
in spiral order.
Example 1:
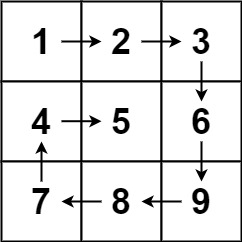
Input: matrix = [[1,2,3],[4,5,6],[7,8,9]] Output: [1,2,3,6,9,8,7,4,5]
Example 2:
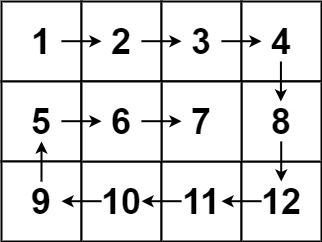
Input: matrix = [[1,2,3,4],[5,6,7,8],[9,10,11,12]] Output: [1,2,3,4,8,12,11,10,9,5,6,7]
Constraints:
m == matrix.length
n == matrix[i].length
1 <= m, n <= 10
-100 <= matrix[i][j] <= 100
Solution
Given an m x n
matrix
, return all elements of the matrix
in spiral order.
/**
* @param {number[][]} matrix
* @return {number[]}
*/
var spiralOrder = function(matrix) {
const res = [];
let i = 0; // row
let j = 0; // col
let offset = 1;
const m = matrix.length;
const n = matrix[0].length;
const size = m * n - 1;
while (res.length < size) {
// right direction
for (; j < n - offset && res.length < size; j++) {
res.push(matrix[i][j]);
}
// down direction
for (; i < m - offset && res.length < size; i++) {
res.push(matrix[i][j]);
}
// left direction
for (; j >= offset && res.length < size; j--) {
res.push(matrix[i][j]);
}
offset++; // increment offset
// up direction
for (; i >= offset && res.length < size; i--) {
res.push(matrix[i][j]);
}
}
res.push(matrix[i][j]);
return res;
};
We keep track of the current row i
, col j
, and the offset offset
relative to the boundary. The code for each of the four directions are self explanatory. Note that the last element in all the directions are appended as the first element of the next direction (eg. the entire first row is appended by the right direction code except the last element; that last element is appended by the down direction code as the first value of the last col). Since the last element can be thought of as the last element for all directions (ie. a 1 x 1
matrix), so non of the directions will append it to res
. Thus, we must manually append matrix[i][j]
after the loop to take care of the last element.