Set Matrix Zeroes
Problem
Given an m x n
integer matrix matrix
, if an element is 0
, set its entire row and column to 0
's, and return the matrix.
You must do it in place.
Example 1:
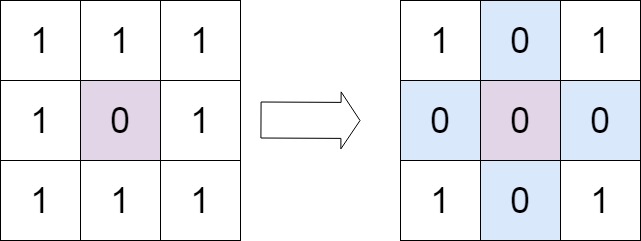
Input: matrix = [[1,1,1],[1,0,1],[1,1,1]] Output: [[1,0,1],[0,0,0],[1,0,1]]
Example 2:
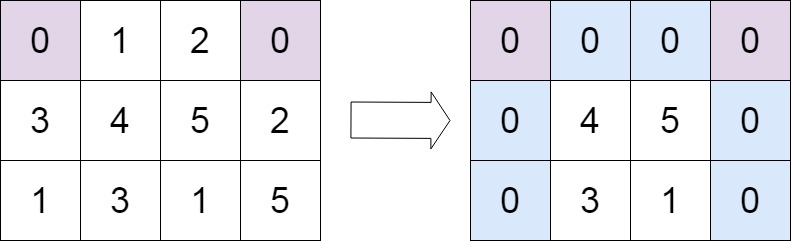
Input: matrix = [[0,1,2,0],[3,4,5,2],[1,3,1,5]] Output: [[0,0,0,0],[0,4,5,0],[0,3,1,0]]
Constraints:
m == matrix.length
n == matrix[0].length
1 <= m, n <= 200
-231 <= matrix[i][j] <= 231 - 1
Solution
/**
* @param {number[][]} matrix
* @return {void} Do not return anything, modify matrix in-place instead.
*/
var setZeroes = function(matrix) {
let col0 = false;
let row0 = false;
const m = matrix.length;
const n = matrix[0].length;
// check if 0th col has any zeroes
for (let i = 0; i < m; i++) {
if (!matrix[i][0]) {
col0 = true;
break;
}
}
// check if 0th row has any zeroes
for (let j = 0; j < n; j++) {
if (!matrix[0][j]) {
row0 = true;
break;
}
}
// check if non 0th rows and cols have any zeroes
for (let i = 1; i < m; i++) {
for (let j = 1; j < n; j++) {
if (!matrix[i][j]) {
matrix[0][j] = 0;
matrix[i][0] = 0;
}
}
}
// set non 0th row of zero entries
for (let i = 1; i < m; i++) {
if (!matrix[i][0]) {
for (let j = 1; j < n; j++) {
matrix[i][j] = 0;
}
}
}
// set non 0th cols of zero entries
for (let j = 1; j < n; j++) {
if (!matrix[0][j]) {
for (let i = 1; i < m; i++) {
matrix[i][j] = 0;
}
}
}
// set 0th cols of zero entries
if (col0) {
for (let i = 0; i < m; i++) {
matrix[i][0] = 0;
}
}
// set 0th col of zero entries
if (row0) {
for (let j = 0; j < n; j++) {
matrix[0][j] = 0;
}
}
};
};
We will use the 0th
column and 0th
row to keep track of whether a row or column needs to be set to all zeroes (eg. if the 0th
row and 6th
column is 0, then this tells us we need to set the entire 6th
column to zeroes). Note that we must first test if the 0th
row or 0th
column have zeroes themselves before any modification.