Remove Nth Node From End of List
Problem
Given the head
of a linked list, remove the nth
node from the end of the list and return its head.
Example 1:
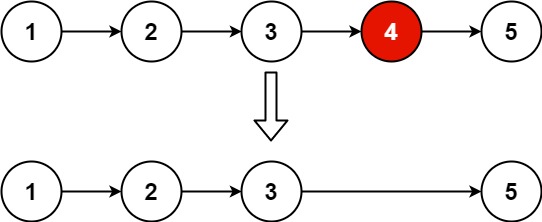
Input: head = [1,2,3,4,5], n = 2 Output: [1,2,3,5]
Example 2:
Input: head = [1], n = 1 Output: []
Example 3:
Input: head = [1,2], n = 1 Output: [1]
Constraints:
- The number of nodes in the list is
sz
. 1 <= sz <= 30
0 <= Node.val <= 100
1 <= n <= sz
Solution
/**
* Definition for singly-linked list.
* function ListNode(val, next) {
* this.val = (val===undefined ? 0 : val)
* this.next = (next===undefined ? null : next)
* }
*/
/**
* @param {ListNode} head
* @param {number} n
* @return {ListNode}
*/
var removeNthFromEnd = function(head, n) {
let fast = head;
let slow = head;
for (let i = 0; i < n; i++) {
fast = fast.next;
}
if (!fast) {
return head.next;
}
while (fast.next) {
fast = fast.next;
slow = slow.next;
}
slow.next = slow.next.next;
return head;
};
We keep track of two pointers, one fast and one slow. The fast pointer will be n
steps ahead of the slow pointer. If after n
iterations the fast pointer becomes null
, then it must mean we need to remove the first node from the list. Otherwise, traverse to the end of the list while updating the fast and slow pointers. When the fast pointer reaches the last element of the list, the slow pointer should be exactly at the position where the next element is the one that needs to be removed (ie. the nth node from the end of the list).